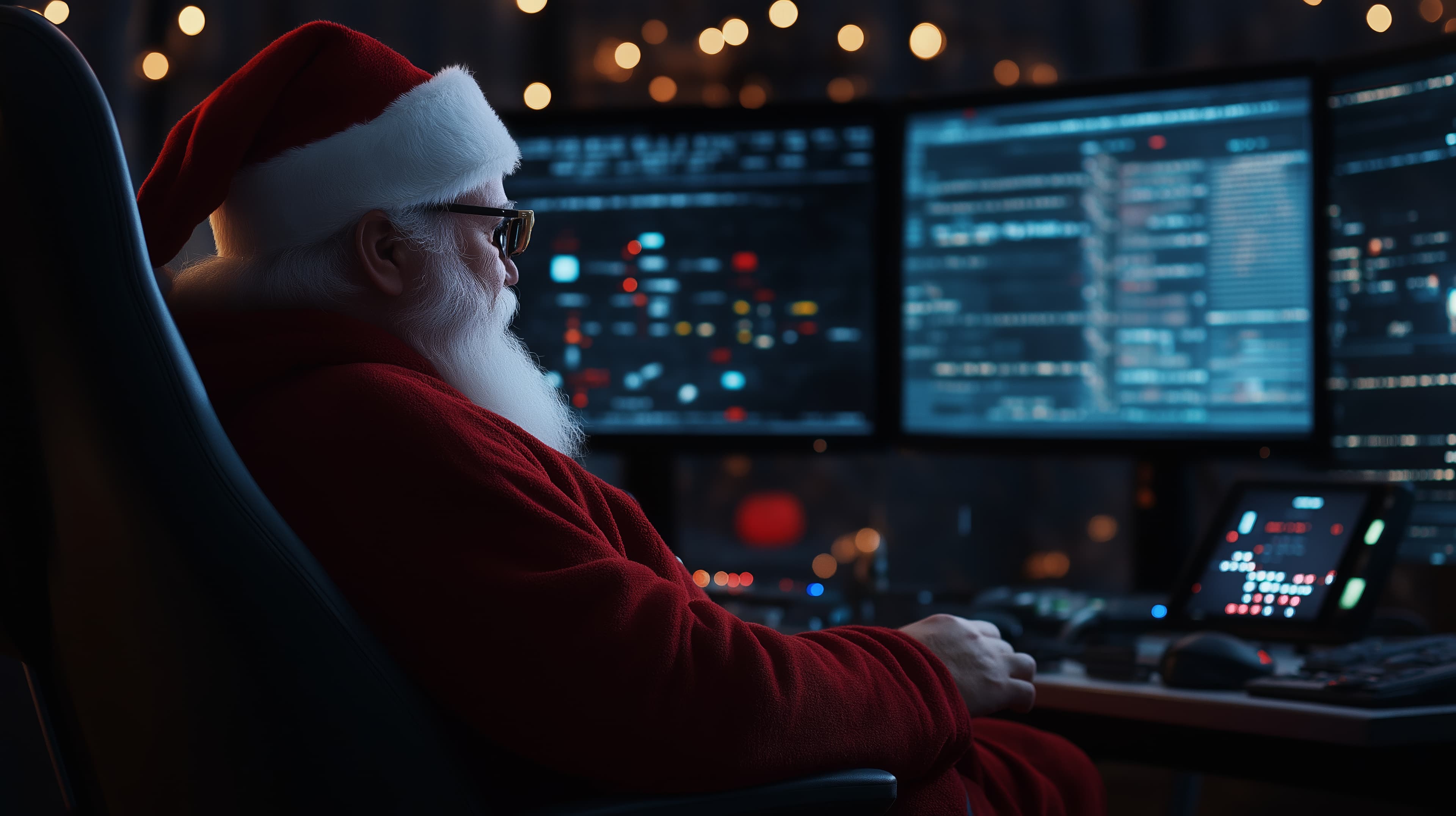
I spent my Christmas holidays being productive and learning new technologies.
My goal was to challenge myself by building a mobile messaging app.
I had previous experience working with React Native and Firebase. This time, I decided to try out Expo instead. Firebase is a NoSQL-based BaaS. There were other options available, like Supabase and Prisma.
I considered Supabase because it was based on a relational database that uses PostgreSQL. I have some experience with MySQL from my university days, so I wanted to try this instead.
Requirements
I knew my mobile app would require authentication so users can register with their login details and navigate based on the auth state.
The user should be able to talk to another user as their friend by sending and receiving requests instantly. They should also be able to change their name and update their status.
Research
When planning the application, I didn't want to spend too much time creating the functionality from scratch. I decided to follow a tutorial just to familiarize myself with the tech stack. I followed a mobile developer's workshop called NotJust.dev on YouTube. This tutorial was to create a pizza/recipe order app which has CRUD functionality. I took the bits that I needed, like authentication and basic CRUD operations, and then did some research to fit my requirements.
Backend Tools
Using Supabase is quite easy; the documentation is very helpful and has many resources available. Supabase uses PostgreSQL as its database, which is a relational database. The automated authentication, encryption, database management, and API generation features make Supabase a powerful and efficient platform for building modern applications.
Overview of application
Here are some shots of my application. I will admit it doesn't look visually appealing, as I didn't spend time designing the user interface.
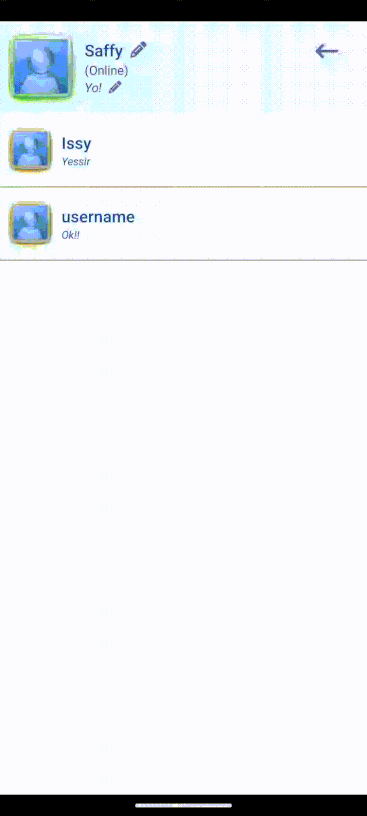
Handling Forms
To handle input forms, I used React's useState
hooks, which allow you to set and get values when needed. We listen to the input value and then store it as a string. This can become tedious if you have many input forms. I decided to use React Hook Form because it handles input validations and improves performance.
Here is how the code looks:
const CustomInput = (props) => {
return (
<Controller
name={name}
control={control}
rules={rules}
render={({
field: { onChange, onBlur, value },
fieldState: { error },
) => (
<>
<View>
<TextInput
placeholder={placeholder}
editable={editable}
onBlur={onBlur}
onChangeText={onChange}
value={value}
secureTextEntry={secureTextEntry}
allowFontScaling={true}
underlineColorAndroid="transparent"
/>
</View>
</>
)}
/>
);
};
React Query
React Query is a powerful data-fetching and state management library for React applications. It simplifies the process of fetching, caching, synchronising, and updating server-state in your React components. Think of it as a super-powered useState
and useEffect
specifically designed for handling data from APIs.
Conclusion
Overall, the development experience was great. The documentation was easy to navigate, and Expo came with tools in the box that I wish to delve deeper into. I will make changes in the future by implementing a notification system to improve user experience. Use encryption methods to obsfucate data for secure messaging.